Data Inspector Control
A data inspector control allows you to view the underlying data for each visible map layer that supports feature queries. It can be configured to function in one of two types of modes: only display when the map is clicked, or real-time streaming data as the user pans over the map.
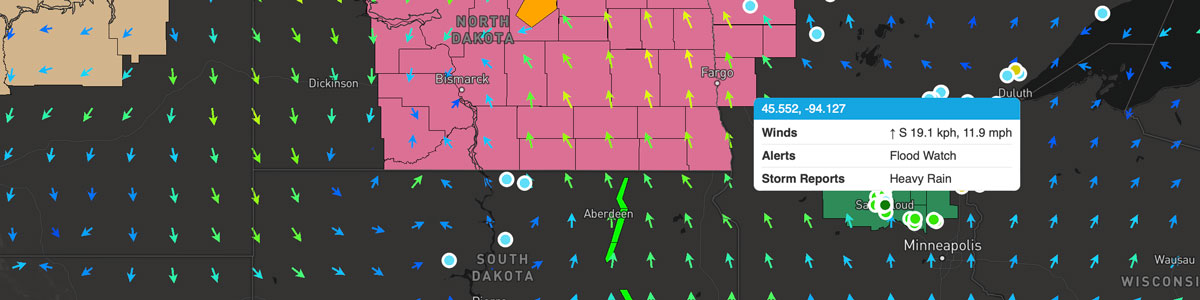
// Adds a data inspector control to the map, returning the control instance
const control = controller.addDataInspectorControl(options);
// Removes the data inspector control
controller.removeDataInspectorControl();
Configuration
The following configuration options are supported when instantiating DataInspectorControl
instances:
Option | Description | Default |
---|---|---|
event | Type: click or move (optional)The event mode for the control:- click: Control will only appear and query data when the map is clicked/tapped. - move: Control will remain visible and continuously query map features and data as the mouse is moved over the map. |
|
stream | Type: boolean (optional)Whether feature queries should happen continuously while map data is animating. If true , then the control will continuously update its values even during animation playback. |
|
Methods
The following methods are available on DataInspectorControl
instances:
addTo(target: HTMLElement | string)
show(point: Point, coord?: Coordinate)
hide()
enable()
disable()
update()
setEvaluator(layerId: string, evaluator: DataEvaluator)
remove()
Data Evaluators
Data evaluators are functions used by a data inspector control in order to format data values returned when querying map features at a specific coordinate. Additionally, you can provide a custom title to display for the layer alongside its data if you don't want to use the default title, which is just the layer's unique identifier.
The MapsGL SDK already includes several built-in data evaluators associated with the available weather layers. However, you may want to override these in order to format the data values differently or provide additional content in the output. There are two ways to set custom data evaluators for layers: including it when adding a weather layer to the map, or afterwards by setting it directly on the data inspector control.
Customizing via Weather Layer Overrides
When you add a weather layer to the map, you can optionally provide a set of overrides to customize the default layer configuration the SDK provides. For example, providing a custom data evaluator for the temperatures
weather layer would be similar to:
const units = aerisweather.mapsgl.units;
controller.addWeatherLayer('temperatures', {
data: {
evaluator: {
title: 'Temperature',
fn: (value) => (
`${value.toFixed(2)}°C, ${units.CtoF(value).toFixed(2)}°F`
)
}
}
});
Note that for the temperatures
layer, value
is a simple float value. However, some layers consist of multiple values, like those related to winds and other vector data. For these layers, the value
passed to your data evaluator function will be an object consisting of two float values: speed
and angle
:
const units = aerisweather.mapsgl.units;
controller.addWeatherLayer('wind-speeds', {
data: {
evaluator: {
title: 'Winds',
fn: (value) => {
const speed = value.speed;
const angle = value.angle - 180;
return `${units.degToDir(angle)} ${units.msToKph(speed).toFixed(1)} kph, ${units.msToMph(speed).toFixed(1)} mph`;
}
}
}
});
Customizing via the Data Inspector Control
Alternatively, you can change a layer's data evaluator after it has already been added to the map by setting it directly on the map's data inspector control. Just use the setEvaluator()
method on the control and provide the unique identifier for the layer you want to update and the data evaluator configuration to want to use:
const control = controller.controls.dataInspector;
const units = aerisweather.mapsgl.units;
control.setEvaluator('temperatures', {
title: 'Temperature',
fn: (value) => (
`${value.toFixed(2)}°C, ${units.CtoF(value).toFixed(2)}°F`
)
});
Similar to the override method described above, setting an evaluator for vector-based data works the same way:
const control = controller.controls.dataInspector;
const units = aerisweather.mapsgl.units;
control.setEvaluator('wind-speeds', {
title: 'Winds',
fn: (value) => {
const speed = value.speed;
const angle = value.angle - 180;
return `${units.degToDir(angle)} ${units.msToKph(speed).toFixed(1)} kph, ${units.msToMph(speed).toFixed(1)} mph`;
}
});