Map Controller
A map controller provides the interface between your map view, which is created using a third-party mapping library such as Leaflet or Mapbox, and the functionality available within the MapsGL SDK. Therefore, you will be working with a map controller instance most of the time when managing data sources and layers or controlling the animation timeline.
To start working with your map, you'll need to create a map controller instance that corresponds to the mapping library you're using. Refer to the list of supported third-party mapping library that MapsGL currently integrates with to determine which map controller you need to instantiate as it is based on your chosen mapping library.
The following examples demonstrate how to set up a map controller using the supported mapping libraries:
// create the Maplibre map instance
const map = new maplibregl.Map({
container: document.getElementById('map'),
style: 'https://api.maptiler.com/maps/streets-v2-light/style.json?key=MAPTILER_KEY',
center: [-74.5, 40],
zoom: 3
});
// create an Account instance with our AerisWeather account access keys
const account = new aerisweather.mapsgl.Account('CLIENT_ID', 'CLIENT_SECRET');
// create a MaplibreMapController instance and provide the above `map` and `account` instances
const controller = new aerisweather.mapsgl.MaplibreMapController(map, { account });
React Usage: If you're using React, check out our react-mapsgl (opens in a new tab) library for quickly integrating MapsGL into your React applications.
Managing Map Data
With a map controller created, you can start adding data to your map. To do so, you can quickly add our pre-configured weather layers to your map without needing to create the data sources and layers directly. Review our guide on using weather layers for more information.
Alternatively, for custom datasets you'll need to set up the appropriate data sources, layers and render styles for your data and visualization requirements. You'll first want to start by adding data sources to your map, so review the data sources guide next for more information.
Map Controls
You can include additional controls to your map controller, such as a legend or real-time data inspector. These controls provide additional functionality related to the map and/or active data layers on the map.
Legend Control
A legend control is responsible for managing the collection of legends currently visible within the context of an interface or map and provides the interface needed for adding and removing legends.
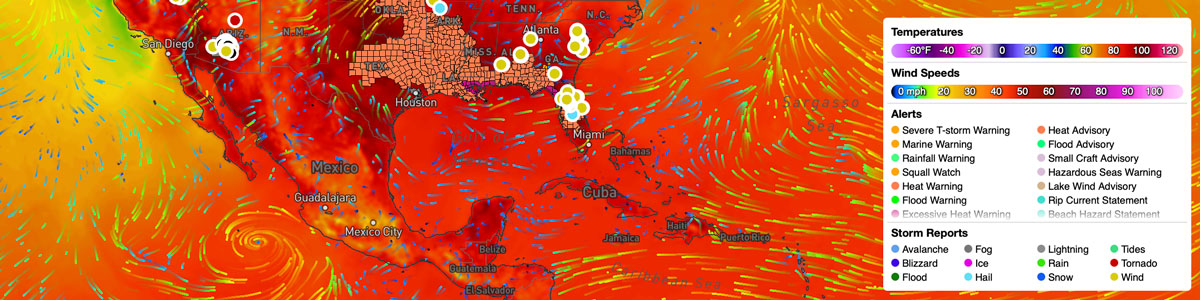
To add a legend control to your map controller, just call addLegendControl()
and specify the DOM target in which you want to insert the legend element. The control instance will be returned when calling this method:
const control = controller.addLegendControl(target, options);
You can also pass a second argument consisting of the desired configuration options for your legend control. See the Legend Control documentation for more information about the supported options.
You can also remove the legend control from your map after it's been added:
controller.removeLegendControl();
Refer to the legend control documentation for more information on configuring and using the control.
Data Inspector Control
A data inspector control allows you to view the underlying data for each visible map layer that supports feature queries. It can be configured to function in one of two types of modes: only display when the map is clicked, or real-time streaming data as the user pans over the map.
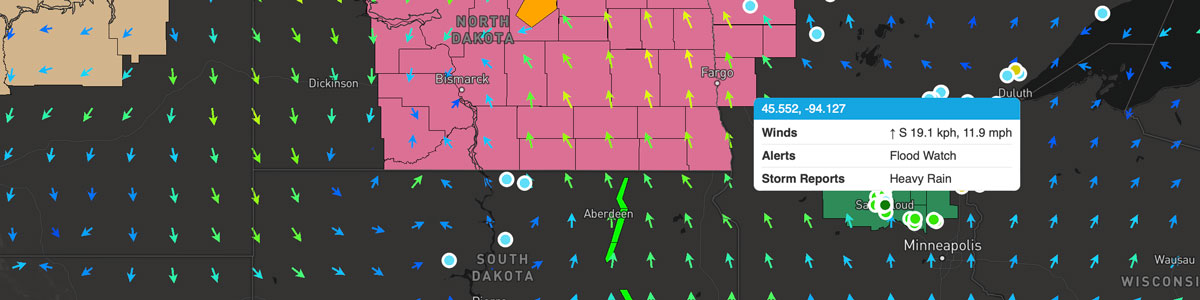
To add a data inspector control to your map controller, just call addDataInspectorControl()
. The control instance will be returned when calling this method:
const control = controller.addDataInspectorControl(options);
You can also pass a second argument consisting of the desired configuration options for your data inspector control. See the Data Inspector Control documentation for more information about the supported options.
You can also remove the data inspector control from your map after it's been added:
controller.removeDataInspectorControl();
Refer to the data inspector control documentation for more information on configuring and using the control.