Javascript SDK Usage
While the MapsGL SDK can be used on its own in conjunction with a third-party mapping library, you may want to take advantage of the various interface controls and elements currently offered by the AerisWeather Javascript SDK (opens in a new tab) and its InteractiveMapApp component (opens in a new tab). The InteractiveMapApp component is a fully-functional, feature-rich interactive map application, which can serve as your own weather dashboard or be integrated into your custom weather applications.
Getting Started
Using the MapsGL SDK with the Javascript SDK works similarly to modules (opens in a new tab) in that the AerisWeather
contains a mapsgl()
method as the entry point for working with MapsGL functionalty.
The mapsgl()
entry point will load the MapsGL SDK assets asynchronously and return a Promise once all required assets have loaded and a MapController
instance has been set up and integrated with your InteractiveMapApp
instance:
// load in MapsGL sdk and set up relevant layer controls
aeris.mapsgl(app, options).then(({ controller, mapsgl }) => {
// "controller" is the MapController instance created for you
// "mapsgl" is the MapsGL SDK public interface if you need to access more MapsGL SDK features
});
At a minimum, you will need to provide your InteractiveMapApp
instance as the first argument when calling aeris.mapsgl()
, which is the app
variable in the above example. You may also pass additional options as the second argument which allows you to further integrate your desired MapsGL layers into your map application's layer control panel.
The following interfaces describe the supported options:
interface MapsGLLayer {
id: string;
title: string;
value: string;
controls: Record<string, any>;
options: Record<string, any>;
index: number;
segments: Array<MapsGLLayer>;
buttons: Array<any>;
}
interface MapsGLMapOptions {
// MapsGL SDK version (uses "latest" by default)
version: string;
// layer panel button configurations for MapsGL-related layers
layers: Array<MapsGLLayer>;
}
The layers
option provides the layer control configuration for your MapsGL-related layers which will get injected into your existing InteractiveMapApp
instance's layers control panel. Refer to the Layers Panel (opens in a new tab) documentation from the Javascript SDK for additional information on the supported properties outlined above.
Example Setup
The following example demonstrates how easy it is to integrate the MapsGL SDK and related weather layers into an InteractiveMapApp. Here we're adding the standard raster satellite
layer and Tropical module (opens in a new tab) from the Javascript SDK along with the default radar
and conditions-related weather layers built into the MapsGL SDK.
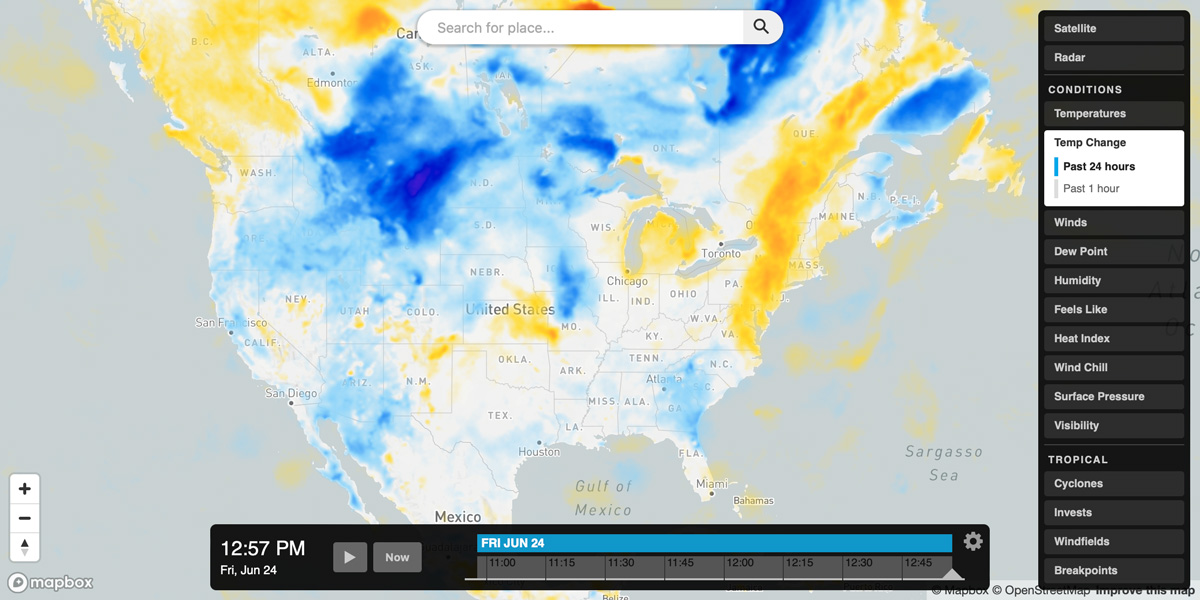
window.addEventListener('load', () => {
const aeris = new AerisWeather('CLIENT_ID', 'CLIENT_SECRET');
aeris.apps().then((apps) => {
const app = new apps.InteractiveMapApp('#map', {
map: {
strategy: 'mapbox',
center: { lat: 40, lon: -90 },
zoom: 4,
map: {
accessToken: 'MAPBOX_TOKEN'
}
},
panels: {
layers: {
buttons: [{
id: 'satellite',
value: 'satellite:75',
title: 'Satellite'
}]
}
}
});
app.on('ready', () => {
// load in MapsGL sdk and set up relevant layer controls
aeris.mapsgl(app, {
layers: [{
title: 'Radar', value: 'radar', options: {
animation: {
interval: 12
}
}
}, {
title: 'Conditions',
buttons: [
{ title: 'Temperatures', value: 'temperatures' },
{ title: 'Temp Change', value: 'temp-change', segments: [
{ title: 'Past 24 hours', value: 'temperatures-24hr-change' },
{ title: 'Past 1 hour', value: 'temperatures-1hr-change' },
] },
{ title: 'Winds', value: 'winds', segments: [
{ title: 'Particles', value: 'wind-particles' },
{ title: 'Fill', value: 'wind-speeds' },
{ title: 'Arrows', value: 'wind-dir' },
{ title: 'Barbs', value: 'wind-barbs' }
] },
{ title: 'Dew Point', value: 'dew-points' },
{ title: 'Humidity', value: 'humidity' },
{ title: 'Feels Like', value: 'feels-like' },
{ title: 'Heat Index', value: 'heat-index' },
{ title: 'Wind Chill', value: 'wind-chill' },
{ title: 'Surface Pressure', value: 'pressure', segments: [
{ title: 'Fill', value: 'pressure-msl' },
{ title: 'Contours', value: 'pressure-msl-contour' },
] },
{ title: 'Visibility', value: 'visibility' },
]
}]
}).then(({ controller, mapsgl }) => {
// add the MapsGL legend control
controller.addLegendControl(app.$el, { width: 300 });
// add the MapsGL data inspector control
controller.addDataInspectorControl();
// add Tropical module from the Javascript SDK
aeris.modules().then((modules) => {
app.modules.add(modules.groups.Tropical);
});
});
});
});
});