Maps Layout
Object
aeris.wxblox.layouts.local.Maps
Data Usage
Raster Maps usage based on size of map and total layers.
The Maps layout displays and manages a MapController view along with a MapListing menu for changing data layers and a map region menu for updating the active bounds for the map.
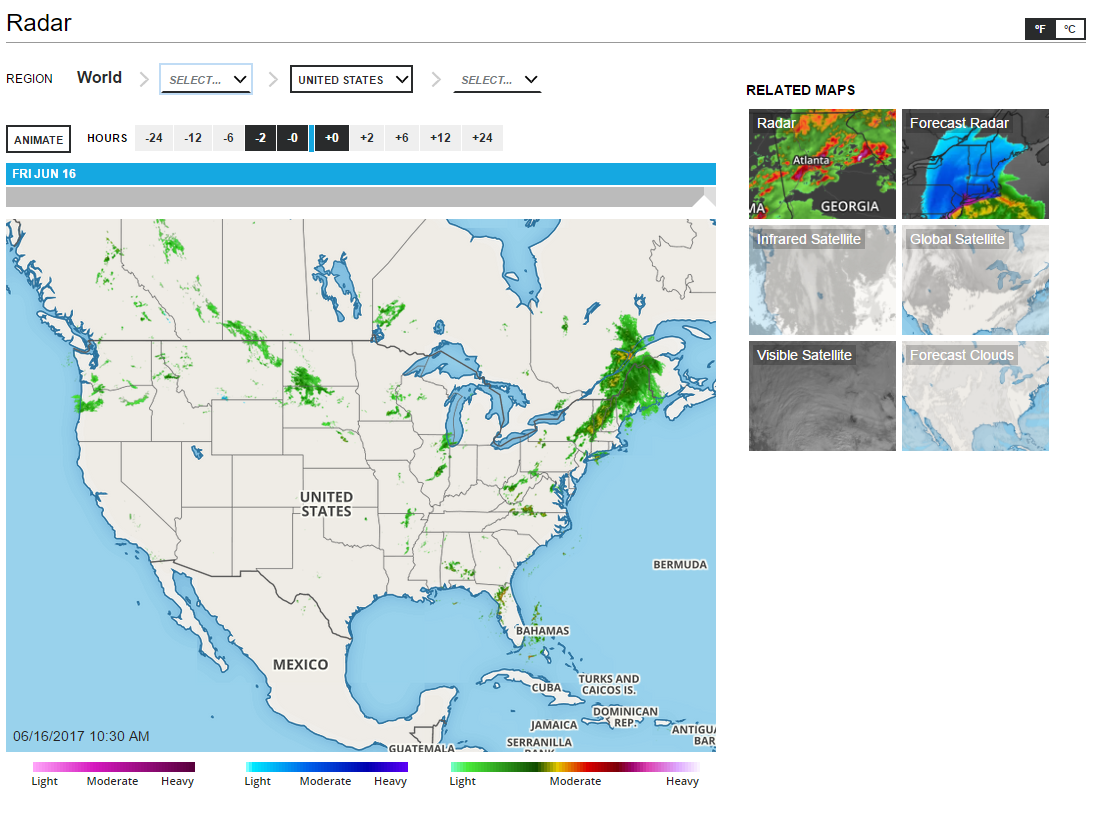
Examples
Display a weather map view using the default configuration with custom options for layers (MapListing) component:
const view = new aeris.wxblox.layouts.Maps('#wxblox', {
layers: {
categories: [],
codes: ['radar', 'alerts', 'temperatures']
}
});
view.load();
Display a weather map view using a custom region selector menu and different categories for the maps listing:
const view = new aeris.wxblox.layouts.Maps('#wxblox', {
layers: {
categories: ['radar/sat', 'severe', 'observations']
},
regions: {
items: {
us: {
label: 'United States'
},
region: {
us: aeris.wxblox.regions.asArray(['usne','usse','usnc','ussc','usnw','ussw'], {
label: 'name',
value: 'code'
})
}
}
}
});
view.load();
Configuration
The following configuration options are supported by this view:
Option | Description | Default |
---|---|---|
controller | Type: object (undefined)Configuration options passed to the internal MapController view. | `` |
controller.viewer | Type: object (undefined)Configuration options passed to the internal Map Viewer view. | `` |
controller.viewer.map | Type: object (undefined)Default map configuration. | `` |
controller.viewer.map.layers | Type: object (undefined)The layer configuration for the map. | `` |
controller.viewer.map.layers.base | Type: array (undefined)An array of base map layer codes to render below all weather and overlay layers. |
|
controller.viewer.map.layers.data | Type: array (undefined)An array of supported weather layer codes. |
|
controller.viewer.map.layers.overlays | Type: array (undefined)An array of overlay layer codes to render above all weather and base layers. |
|
controller.viewer.map.size | Type: object (undefined)Configuration for the map size. |
|
controller.viewer.map.size.width | Type: number (undefined)[integer/auto] Map width in pixels (limited by your Aeris Maps level), or auto to have the width automatically set to fill the map container's width. |
|
controller.viewer.map.size.height | Type: number (undefined)Map height in pixels (limited by your Aeris Maps level), or auto to have the height automatically calculated based on the map container's width and the specified map.size.factor ratio |
|
controller.viewer.map.size.factor | Type: number (undefined)The scale factor to use when height is set to auto . The map height will be calculated by multiplying either the specified width value or DOM container's width by the factor value. |
|
controller.viewer.animation | Type: object (undefined)Configuration for the map animation | `` |
controller.viewer.animation.enabled | Type: boolean (undefined)Whether or not to enabled animation. If false , then the viewer will only display data for the current time |
|
controller.viewer.animation.autoplay | Type: boolean (undefined)Whether or not to have your animation autoplay when the WxBlox layout loads. |
|
controller.viewer.animation.alwaysShowPast | Type: boolean (undefined)Whether or not to display past layers (observed) even if the timeline is in the future. |
|
controller.viewer.animation.alwaysShowFuture | Type: boolean (undefined)Whether or not to display future layers even if the timeline is in the past. |
|
controller.viewer.animation.duration | Type: number (undefined)Total duration of the animation in seconds; duration and intervals are both used to control the overall speed of the animation |
|
controller.viewer.animation.endDelay | Type: number (undefined)Number of seconds to lapse before the animation starts over. |
|
controller.viewer.animation.intervals | Type: number (undefined)Total number of frames to use for the animation; duration and intervals are both used to control the overall speed of the animation. |
|
controller.viewer.overlays | Type: object (undefined)Configuration for custom overlays rendered above the map. | `` |
controller.viewer.overlays.timestamp | Type: string (undefined)[string] or [object] A string value indicates the time and date format for the map's timeline during playback. Provide an object to include additional options. |
|
controller.viewer.overlays.branding | Type: object (undefined)Map branding options. | `` |
controller.viewer.overlays.branding.img | Type: string (undefined)A URL to an image to display on the map, such as a company logo. |
|
controller.viewer.overlays.branding.html | Type: string (undefined)An HTML string to display for the map branding, such as a company logo or name. |
|
controller.range | Type: object (undefined)Configuration for your timeline range. | `` |
controller.range.intervals | Type: string (undefined)Clickable intervals for your animation timeline. |
|
controller.range.init | Type: object (undefined)Default configuration for the initial values of the timeline range. | `` |
controller.range.init.from | Type: number (undefined)Initial from value that is selected for the animation when your WxBlox layout loads. |
|
controller.range.init.to | Type: number (undefined)Initial to value that is selected for the animation when your WxBlox layout loads. |
|
regions | Type: object (undefined)An object consisting of a series of items to display in the region selector menu. Refer to the Region Menu page for usage details. |
|
layers | Type: object (undefined)Configuration options passed to the internal MapListing view. | `` |
layers.categories | Type: string (undefined)Add categories of layers in the Related Maps section. Applicable categories: |
|
layers.codes | Type: string (undefined)Custom list of layers based on layer name. |
|
layers.type | Type: string (undefined)"list" or "thumbs" to display the format of related maps. Thumbs will be the thumbnails while list will be text. |
|
layers.links | Type: boolean (undefined)Determines whether or not the component should use links when an item is selected or trigger an event. If set to false , you are responsible for updating your page and/or map content based on the selected item in the listing. |
|
selectors | Type: object (undefined)Selectors for DOM render targets | `` |
selectors.controller | Type: string (undefined)DOM target where the internal MapController component is rendered |
|
selectors.regions | Type: string (undefined)DOM target where the region selector menu is rendered |
|
selectors.layers | Type: string (undefined)DOM target where the internal MapListing component is rendered |
|
init | Type: object (undefined)The initial values when the component is rendered | `` |
init.regions | Type: object (undefined)The initially-selected value in the region selector menu |
|
init.regions.key | Type: string (undefined)Regional category that will be initially selected when WeatherBlox component loads. |
|
init.layers | Type: object (undefined)The initially-selected value in the map layers listing menu |
|
init.layers.value | Type: string (undefined)AMP layer that will be initially selected when WxBlox component loads. |
|
enabled | Type: boolean (undefined)Whether or not the view is enabled. If false then the view will not be rendered and data required for the view will not be requested. This option is typically only applicable for views contained within a parent layout. |
|
metric | Type: boolean (undefined)Whether or not to display units in metric. The method setUnits() can be used at runtime once a view has rendered to change the units currently displayed. |
|
Methods
The following methods are supported by instances of this view:
rendered()
show()
hide()
params()
setUnits(:number)
units()
enabled()
setMetric(:boolean)
load(:object)
refresh()
isMetric()
Events
The following events are triggered by instances of this view:
render
render:before
render:after
load:start
load:done
load:error
change:units