Map Viewer
Object
aeris.wxblox.views.MapViewer
Data Usage
Raster Maps usage based on size of map and total layers.
A MapViewer view is responsible for displaying and managing a weather map consisting of one or more data layers. This view also handles animating weather data across a specific time range.
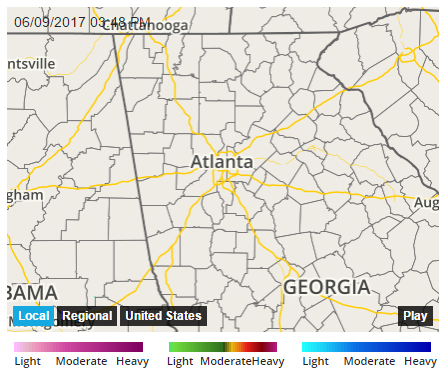
Examples
Display a MapViewer component with navigation options to change the zoom level and layer data types centered on Austin, TX:
const view = new aeris.wxblox.views.MapViewer('#wxblox', {
map: {
loc: 'austin,tx',
data: ['radar']
},
controls: {
layers: [{
value: 'radar',
title: 'Radar'
},{
value: 'satellite',
title: 'Satellite'
},{
value: 'alerts',
title: 'Advisories'
},{
value: 'temperatures,clip-us-flat',
title: 'Temps'
}],
regions: [{
zoom: 7,
title: 'Local'
},{
zoom: 5,
title: 'Regional'
},{
region: 'us',
title: 'National'
}]
}
});
view.load();
Configuration
The following configuration options are supported by this view:
Option | Description | Default |
---|---|---|
map | Type: object (undefined)Default map configuration | `` |
map.layers | Type: object (undefined)The layer configuration for the map | `` |
map.layers.base | Type: array (undefined)An array of base map layer codes to render below all weather and overlay layers |
|
map.layers.overlays | Type: array (undefined)An array of overlay layer codes to render above all weather and base layers |
|
map.layers.data | Type: array (undefined)An array of supported weather layer codes |
|
map.center | Type: string (undefined)The location to center the map on |
|
map.zoom | Type: number (undefined)Map zoom level |
|
map.bounds | Type: string (undefined)[string] or [object] The bounds to use for the map as northwest and southeast coordinates either as a string, 56.26,-131.39,15.45,-61.17 , or object, { north: 56.26, west: -131.39, south: 15.45, east: -61.17 } |
|
map.region | Type: string (undefined)The key of a supported region to display for the map. See the regions documentation for the regions currently supported and their usage. |
|
map.size.width | Type: number (undefined)[integer/auto] Map width in pixels (limited by your Aeris Maps level), or auto to have the width automatically set to fill the map container's width. |
|
map.size.height | Type: number (undefined)[integer/auto] Map height in pixels (limited by your Aeris Maps level), or auto to have the height automatically calculated based on the map container's width and the specified map.size.factor ratio |
|
map.size.factor | Type: number (undefined)The scale factor to use when height is set to auto . The map height will be calculated by multiplying either the specified width value or DOM container's width by the factor value. |
|
map.offset | Type: string (undefined)The time offset to display for single-interval maps. This does not affect animations |
|
map.autoFuture | Type: boolean (undefined)Whether or not future layers corresponding to active layers defined in map.layers.data should automatically be included when the map's timeline includes future periods (e.g. fradar would be included if radar is active) |
|
map.combine | Type: boolean (undefined)Whether or not all specified layers specified by map.layers.data should be combined into a single image for each request. When false , the base overlay layers will be rendered into their own separate DOM containers below and above the map content respectively and will not be animated, which reduces the total map units used for a single animation. Set to true if you need your layers rendered in a unique order around the animated map layers. |
|
animation | Type: object (undefined)Configuration for the map animation | `` |
animation.enabled | Type: boolean (undefined)Whether or not to enabled animation. If false , then the viewer will only display data for the current time |
|
animation.from | Type: number (undefined)[number] or [date] Start time offset relative to now for the animation in seconds, or a valid Date instance; negative values are in the past |
|
animation.to | Type: number (undefined)End time offset relative to now for the animation in seconds, or a valid Date instance; negative values are in the past |
|
animation.duration | Type: number (undefined)Total duration of the animation in seconds; duration and intervals are both used to control the overall speed of the animation |
|
animation.endDelay | Type: number (undefined)Delay to hold the end of the animation before replaying from the beginning, in seconds |
|
animation.intervals | Type: number (undefined)Total number of frames to use for the animation; duration and intervals are both used to control the overall speed of the animation such that a higher number of intervals will animate faster than a lower number of intervals for the same duration value |
|
animation.autoplay | Type: boolean (undefined)Whether or not the animation should automatically start playing initially |
|
animation.refresh | Type: number (undefined)How often the animation re-fetches data. |
|
overlays | Type: object (undefined)Configuration for custom overlays rendered above the map | `` |
overlays.title | Type: string (undefined)he title to display over the map. Use CSS to style the title's DOM elements as needed |
|
overlays.timestamp | Type: string (undefined)[string] or [object] A string value indicates the time and date format for the map's timeline during playback. Provide an object to include additional options. |
|
overlays.timestamp.format | Type: string (undefined)Time and date format for the map timeline during playback using moment.js formatting options |
|
overlays.timestamp.continuous | Type: boolean (undefined)Whether or not the timestamp should update continuously during playback regardless of frame intervals. If false , then the timestamp will only update when the animation's frame changes |
|
overlays.branding | Type: object (undefined)Map branding options | `` |
overlays.branding.img | Type: string (undefined)A URL to an image to display on the map, such as a company logo. |
|
overlays.branding.html | Type: string (undefined)An HTML string to display for the map branding, such as a company logo or name. Offers more flexible customization options than overlays.branding.img . |
|
selectors | Type: object (undefined)Selectors for DOM render targets | `` |
controls | Type: object (undefined)Options for layers and regions navigation elements displayed within the viewer | `` |
controls.layers | Type: array (undefined)An array of layer config objects to display in the layer selection navigation |
|
controls.layers[#].value | Type: string (undefined)The AerisMaps layer code | `` |
controls.layers[#].title | Type: string (undefined)The title to display for the layer | `` |
controls.regions | Type: array (undefined)An array of region config objects to display in the region selection navigation |
|
controls.regions[#].zoom | Type: number (undefined)The map zoom level to use for the region | `` |
controls.regions[#].loc | Type: string (undefined)An optional location to center the map on for the region. If this value is not defined or null , then the value of map.loc will be used for this region's center location. | `` |
controls.regions[#].title | Type: string (undefined)The title to display for the region | `` |
enabled | Type: boolean (undefined)Whether or not the view is enabled. If false then the view will not be rendered and data required for the view will not be requested. This option is typically only applicable for views contained within a parent layout. |
|
metric | Type: boolean (undefined)Whether or not to display units in metric. The method setUnits() can be used at runtime once a view has rendered to change the units currently displayed. |
|
renderNoData | Type: boolean (undefined)Whether or not the view should be rendered if data was not returned or not available. |
|
request | Type: object (undefined)An object containing the default Weather API request options to use for the views's data request where applicable. These parameters can also be overriden in the load(:params) method when rendering the view using the JavaScript method. | `` |
Methods
The following methods are supported by instances of this view:
viewer()
rendered()
show()
hide()
params()
setUnits(:number)
units()
enabled()
setMetric(:boolean)
load(:object)
refresh()
isMetric()
Events
The following events are triggered by instances of this view:
render
render:before
render:after
load:start
load:done
load:error
change:units